invert binary tree iterative
We can ask ourselves which tree traversal would be best to invert the binary tree. If root current node is NULL inverting is done.
Python Inverting Binary Tree Recursive Stack Overflow
Insert the left and right child into the queue.
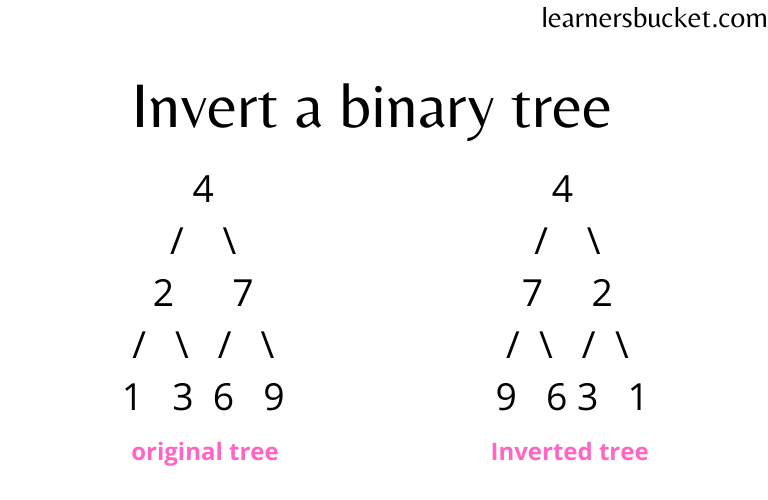
. We can literally solve this problem recursively with these three lines of code. The inverted tree will. In this tutorial I am.
Invert Binary Tree. So you can invert the tree by inverting the predicate -- eg instead of testing for a b test for a b. Write a function that takes in a Binary Tree and inverts it.
In other words the function should swap every left node in the tree for its corresponding right. Swap left and right child of node N. To invert the tree iteratively Perform the level order traversal using the queue.
Suppose we have a binary tree. We dont need to change the structure of the tree at all to invert it -- we can. Definition for a binary tree node.
The first stack gets the reverse Post. Root 21. Take a look at the below python code to understand how to invert a binary tree using level order traversal.
Define a queue Q. When the interviewer at Google asks you to. Here are the steps.
Inverting a binary tree means we have to interchange the left and right children of all non-leaf nodes. Root 4271369 Output. Answer 1 of 5.
Given the root of a binary tree invert the tree and return its root. Because rooted trees are recursive data structures algorithms on trees are most naturally expressed. So what is an inverted binary tree.
The inversion of a binary tree or the invert of a binary tree means to convert the tree into its Mirror image. Keep the previous pointer of. Our task is to create an inverted binary tree.
In simple words Output is the mirror of the input tree. Show activity on this post. Def __init__ self val0 leftNone.
The key insight here is to realize that in order to invert a binary tree we only need to swap the children and recursively solve the two smaller sub. Pop node N from queue Q from left side. In mathematics an iterated binary operation is an extension of a binary operation on a set S to a function on finite sequences of elements of S through repeated application.
We can easily convert the above recursive solution into an iterative one using a queue また stack to store tree nodes. Time Space Complexities. It is to be noted that new keys are always inserted at the leaf node.
I was working on a problem Invert Binary Tree in an iterative fashion. Invert Binary Tree. 90 of our engineers use the software you wrote Homebrew but you cant invert a binary tree on a whiteboard so f off - Max Howell Problem definition.
The above recursive solution can be converted to iterative one by using queue or stack to store the nodes of the tree. Steps to invert a Binary Tree iteratively using Queue. Inorder traversal of the constructed tree is 4 2 5 1 3 Inorder traversal of the mirror tree is 3 1 5 2 4.
Given the root of a binary tree invert the tree and return its root. In simple terms it is a tree whose left children and right children of all non-leaf. Add root node to queue Q.
Worst-case Time complexity is On and. 8327 112 Add to List Share. Space Complexity of Invert a Binary Tree.
The Post-Order iterative tree traversal algorithm is slightly more complex than the Pre-Order one because the solution uses two stacks. Store the root node in the queue and then. The code is almost similar to the レベル.
Classic examples are computing the size the height or the sum of values of the tree. In this tutorial I have explained how to invert binary tree using iterative and recursive approachLeetCode June Challenge PlayList - httpswwwyoutubeco. Python Server Side Programming Programming.
Often seen many times in the ad of AlgoExpert as well. So if the tree is like below. Our solution would be recursive.
Preorder is a pretty simple and readable solution. Start from root and run a loop until a null pointer is reached. That will be the only time it is required.
Inverting a binary is one of the most common questions being asked these days. While queue Q is not empty.
Invert A Binary Tree Python Code With Example Favtutor
Leetcode Invert Binary Tree Solution Explained Java Youtube
Invert A Binary Tree Recursive And Iterative Solutions Learnersbucket
Invert A Binary Tree Python Code With Example Favtutor
Invert Reverse A Binary Tree 3 Methods
Invert Binary Tree Iterative Recursive Approach
Invert A Binary Tree Recursive And Iterative Approach In Java The Crazy Programmer
Invert Binary Tree Leetcode 226 Iterative Recursive Approach Youtube
Invert Alternate Levels Of A Perfect Binary Tree Techie Delight
Invert Binary Tree Leetcode 226 Youtube
Invert Binary Tree Iterative And Recursive Solution Techie Delight
Invert A Binary Tree Python Code With Example Favtutor
Invert A Binary Tree Python Code With Example Favtutor
Invert Alternate Levels Of A Perfect Binary Tree Techie Delight
Invert Reverse A Binary Tree 3 Methods
Coding Short Inverting A Binary Tree In Python By Theodore Yoong Medium
Algodaily Software Interview Prep Made Easy Coding Interview Questions
Invert A Binary Tree Bst Carl Paton There Are No Silly Questions